type
status
date
slug
summary
tags
category
icon
password
参数应用集成
导入sdk依赖
加上权限
具体操作
首先需要在开发者中心创建一个应用
获取应用的key和密钥
在代码中集成sdk,并且初始化
在根application中初始化连接,这里就需要key和密钥
接收推送的参数文件,由于推送的参数文件是由maxstore唤起所以有两种一种是广播唤起,还有一种是aidl直接唤起service。这里使用的是service,首先需要创建一个service来写下载的逻辑。
别忘记注册,这里注册比较特殊,需要指定这个?(不太清楚)
到这里,一个可以接收参数的应用就写完了,如何接收呢?
接收ActivityDemo
通过parseDownloadParamXml()方法解析成hashmap,还有解析json的方法(未写)详情可以看这。
推送参数应用的参数(验证)
首先
需要将这个app上架,并设置为参数应用设置参数模板。测试机子需要安装maxstore,并注册。
参数模板
在开发者中心→平台服务→自定义参数模板创建(注意此为创建xml,与最后解析的文件无关,最后推送的文件将由maxstore解析后生成另一个xml文件,也不必过多关心),可导入以下例子参考,需要注意Files里面的
<FileName>
就是文件最后的名字,这里没写.xml后缀,所以生成的文件也不带后缀,这是失误,需要改正,还是规范加上后缀,方便自己拿取的参数文件。<PID>
是参数的键, <Defaultvalue>
是参数的值,通过api可以转换存在hashmap里。准备好参数后就需要推参数了
进入管理员界面通过应用的申请的app,找到需要测试的机子选择应用和应用参数,激活推送。接着就看有没有接收成功了
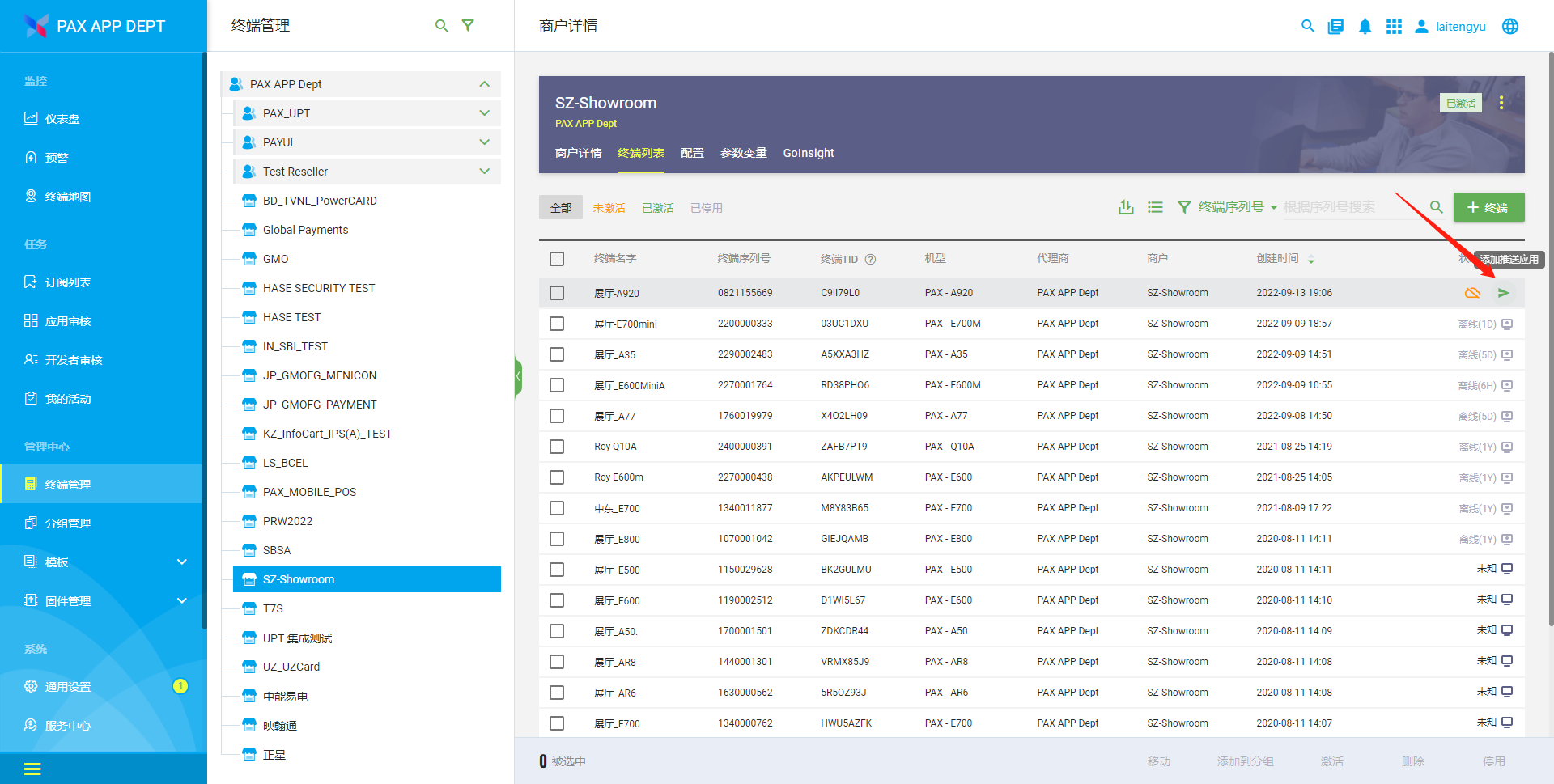
在此修改参数

激活并推送
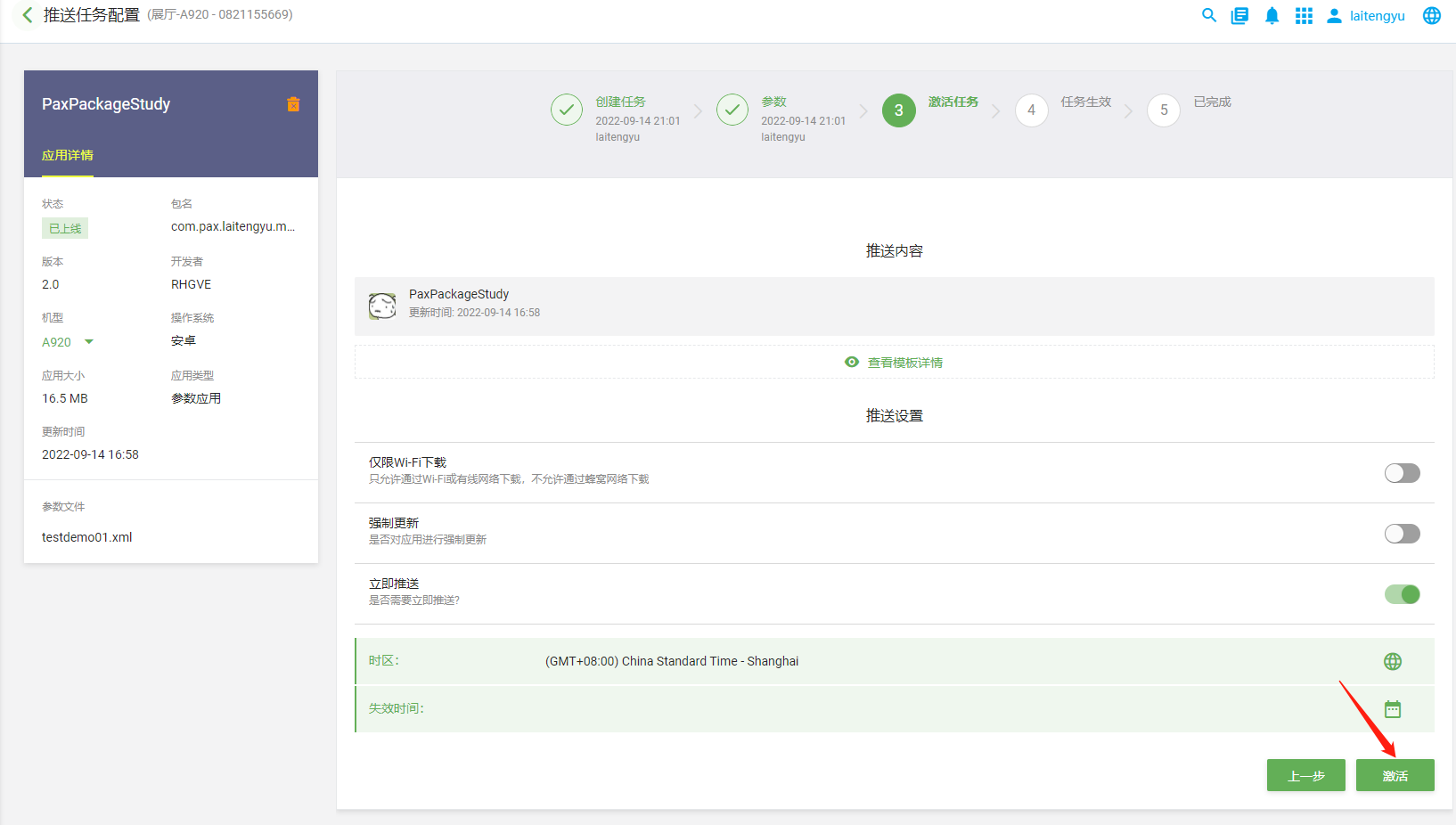
- Author:内河大魔王
- URL:https://ltyzqhh.top/article/%E5%AD%A6%E4%B9%A0maxstore
- Copyright:All articles in this blog, except for special statements, adopt BY-NC-SA agreement. Please indicate the source!